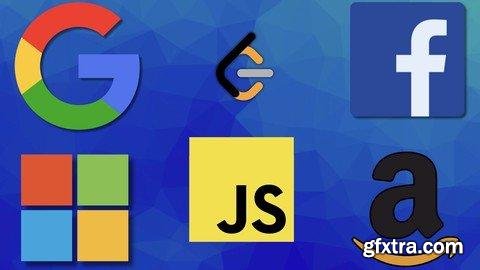
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz
Language: English | Size: 5.40 GB | Duration: 22h 41m
Practice data structure and algorithms questions for interviews at FAANG companies like Google, Facebook, Apple & Amazon
What you'll learn
Solve Easy to Hard Difficulty problems using different data structures and algorithms
How to solve some of the most popular interview questions asked by major tech companies
Breaking down the coding interview problems in a step by step, systematic manner
Popular problems patterns
Algorithms and data structures
Requirements
Basic Knowledge of javascript is preferred
Basic Knowledge of Fundamental data structures and algorithms is preferred
Description
Want to master popular problem-solving techniques, data structures, and algorithms that interviewers love? Dive right in!Crave step-by-step explanations for the industry's hottest interview questions? We've got you covered.Looking to up your game in competitive programming? Buckle up for a thrilling journey!Welcome to the course!In this course, you'll have a detailed, step by step explanation of hand-picked LeetCode questions where you'll learn about the most popular techniques and problems used in the coding interview, This is the course I wish I had when I was doing my interviews. and it comes with a 30-day money-back guaranteeWhat is LeetCode?LeetCode is essentially a huge repository of real interview questions asked by the most popular tech companies ( Google, Amazon, Facebook, Microsoft, and more ).The problem with LeetCode is also its advantage, IT'S HUGE, so huge in fact that interviewers from the most popular companies often directly ask questions they find on LeetCode, So it's hard to navigate through the huge amount of problems to find those that really matter, this is what this course is for.I spent countless hours on LeetCode and I'm telling you that you don't have to do the same and still be able to get a job at a major tech company.Course overview :In this course, I compiled the most important and the most popular interview questions asked by these major companies and I explain them, in a true STEP BY STEP fashion to help you understand exactly how to solve these types of questions.The problems are handpicked to ensure complete coverage of the most popular techniques, data structures, and algorithms used in interviews so you can generalise the patterns you learn here on other problems.Each problem gets multiple videos :Explanation and intution video(s): we do a detailed explanation of the problems and its solution, this video will be longer because we will do a step by step explanation for the problems.Coding video(s): where we code the solution discussed in the explanation video together.Walkthrough video(s): where we go over each line of code and see what it doesWe will use basic javascript for this course to code our solutions, previous knowledge in javascript is preferred but NOT required for the coding part of the course.The problems are categorised for easier navigation and will be regularly updated with more popular and interesting problems.Some of the stuff this course will cover are :Arrays and Strings interview questions.Searching interview questions and algorithms.Dynamic Programming interview questions.Backtracking interview questions ( With step by step visualisation ).Trees and Graphs interview questions and algorithms.Data structures Like Stacks, Queues, Maps, Linked Lists, and more.In other words, this course is your one-stop-shop for your dream job.
Overview
Section 1: Microsoft Array Question: Container with most water (Medium)
Lecture 1 Introduction to the problem
Lecture 2 Brute Force solution Intuition
Lecture 3 pseudocode walkthrough
Lecture 4 Better Approach intuition
Lecture 5 Approach 2 Pseudocode walkthrough
Lecture 6 Implementing the code
Section 2: Google Array Question: Valid mountain array (Easy)
Lecture 7 Introduction to the problem
Lecture 8 How to think about this problem
Lecture 9 Pseudocode Walkthrough
Lecture 10 Implementing the code
Section 3: Google Array Question: Boats to save people (Medium)
Lecture 11 Problem Introduction
Lecture 12 How to intuitively think about this problem
Lecture 13 Pseudocode Walkthrough
Lecture 14 Implementing the code
Section 4: Facebook Array Question: Move Zeroes (Easy)
Lecture 15 Brute force Intuition
Lecture 16 Brute force pseudocode walkthrough
Lecture 17 Better Approach Intuition
Lecture 18 Better Approach Pseudocode walkthrough
Lecture 19 Implementing the code
Section 5: Amazon Array Question: Longest substring without repeating characters (Medium)
Lecture 20 Introduction to the problem
Lecture 21 Brute Force Intuition
Lecture 22 Pseudocode walkthrough
Lecture 23 Approach 2 Intuition
Lecture 24 Approach 2 pseudocode walkthrough
Lecture 25 Implementing the code
Section 6: Arrays Question:Find first and last position of element in sorted Array (Medium)
Lecture 26 Introduction to the problem and brute force approach
Lecture 27 Brute force Pseudocode walkthrough
Lecture 28 Approach 2: Optimal Approach intuition
Lecture 29 Pseudocode walkthrough part 1
Lecture 30 Pseudocode walkthrough part 2
Lecture 31 Implementing the code
Section 7: Google Array question: first bad version (Easy)
Lecture 32 Introduction To The Problem And Brute Force Approach
Lecture 33 Optimal Solution Intuition
Lecture 34 Optimal solution pseudocode walkthrough
Lecture 35 Implementing the code
Section 8: Microsoft Math Question: Missing Number (Easy-ish)
Lecture 36 Introduction to the problem
Lecture 37 Approach 1: Brute Force Approach
Lecture 38 Approach 2: A Better Approach Explanation
Lecture 39 PseudoCode Walkthrough For Approach 2
Lecture 40 Implementing the code
Lecture 41 Approach 3: Optimal Approach
Lecture 42 Implementing the optimal approach
Section 9: Amazon Math Question: Count Primes
Lecture 43 Problem Introduction And Brute Force Explanation
Lecture 44 Pseudocode Walkthrough For Brute Force Approach
Lecture 45 Approach 2: Optimal solution
Lecture 46 Pseudocode Walkthrough For Optimal Approach
Lecture 47 Code Implementation
Section 10: Airbnb Math Question: Single Number
Lecture 48 Introduction to the problem and brute force approach
Lecture 49 Pseudocode walkthrough for brute approach
Lecture 50 Approach 2: better Approach
Lecture 51 Implementing the code
Lecture 52 Approach 3: optimal approach
Lecture 53 Implementing the optimal approach
Section 11: Amazon Math Question: Robot return to origin (Easy)
Lecture 54 Explaining the problem
Lecture 55 Implementing the code
Section 12: Facebook Math Question: Add Binary (Easy)
Lecture 56 Introduction to the problem
Lecture 57 Examples of binary additions
Lecture 58 Pseudocode Implementation
Lecture 59 Pseudocode Walkthrough
Lecture 60 Implementing the code
Section 13: Google Hash Tables / Dictionaries question: Two Sum (Easy)
Lecture 61 Approach 1: Introduction to the problem and brute force approach
Lecture 62 Brute force Pseudocode Implementation
Lecture 63 Pseudocode Walkthrough
Lecture 64 Approach 2: Optimal Approach Explanation
Lecture 65 Pseudocode Walkthrough
Lecture 66 Code Implementation
Section 14: Google Hash Tables / Dictionaries question: Contains Duplicate
Lecture 67 Introduction to the problem and multiple approaches
Lecture 68 Optimal Approach
Lecture 69 Implementing the code
Section 15: Google Hash Tables / Dictionaries question: Majority Element
Lecture 70 Approach 1 Intuition
Lecture 71 Approach 1 Pseudocode Walkthrough
Lecture 72 Approach 2 - Majority Element Intuition
Lecture 73 Implementing Approach 2 code
Lecture 74 Approach 3 Intuition - Optimal solution
Lecture 75 Approach 3 Walkthrough - Optimal solution
Lecture 76 Implementing Approach 3 (optimal approach)
Section 16: Facebook Hash Tables / Dictionaries question: Group Anagrams (Medium)
Lecture 77 Explanation - Group Anagrams - Medium #49
Lecture 78 Code - Group Anagrams - Medium #49
Section 17: Hash Tables / Dictionaries question: 4sum 2 (Medium)
Lecture 79 Brute force Explanation
Lecture 80 Brute Force Pseudocode Walkthrough
Lecture 81 Approach 2: Optimal approach
Lecture 82 Implementing the code
Section 18: Microsoft Hash Tables / Dictionaries question: LRU Cache (Medium)
Lecture 83 Introduction to the problem
Lecture 84 Input/Ouput for the problem
Lecture 85 Intuition behind the problem
Lecture 86 Pseudocode implementation
Lecture 87 Pseudocode Walkthrough
Section 19: Linkedin Hash Tables / Dictionaries question: Minimum Window Substring (Hard)
Lecture 88 Explanation - Minimum Window Substring - part 1
Lecture 89 Explanation - Minimum window substring - part 2
Lecture 90 Explanation - Minimum window substring - part 3
Lecture 91 Pseudocode Implementation
Lecture 92 Pseudocode Walkthrough
Lecture 93 Code - Minimum Window Substring - Hard #76
Section 20: Apple Linked list question: Merge Two Sorted Lists (Easy)
Lecture 94 Explanation - Merge Two Sorted Lists - Easy #21
Lecture 95 Pseudocode Implementation - Merge Two Sorted Lists - Easy #21
Lecture 96 Walkthrough - Merge Two Sorted Lists - Easy #21
Lecture 97 Code - Merge Two Sorted Lists - Easy #21
Section 21: Amazon Linked list question: Linked list cycle (Medium)
Lecture 98 Explanation - Linked List Cycle - Easy #141
Lecture 99 Intuition - Linked List Cycle - Easy #141
Lecture 100 Walkthrough - Linked List Cycle - Easy #141
Lecture 101 Code - Linked List Cycle - Easy #141
Section 22: Microsoft Linked list question: Reverse linked list (Medium)
Lecture 102 Explanation - Reverse Linked List
Lecture 103 Intuition - Reverse Linked List
Lecture 104 pseudocode Implementation & Walkthrough - Reverse Linked List
Lecture 105 Implementing the code - Reverse Linked List
Section 23: Adobe Linked list question: Add two numbers (Medium)
Lecture 106 Explanation - Add Two Numbers
Lecture 107 Intuition - Add Two Numbers
Lecture 108 Pseudocode Implementation - Add Two Numbers
Lecture 109 Walkthrough - Add Two Numbers - Medium #2
Lecture 110 Code - Add Two Numbers
Section 24: Linked list question: Remove Nth node from end of list (Medium)
Lecture 111 Explanation - Remove Nth Node From End of List
Lecture 112 Intuition - Remove Nth Node From End of List
Lecture 113 Walkthrough - Remove Nth Node From End of List
Lecture 114 Approach 2 Explanation - Remove Nth Node From End of List
Lecture 115 Approach 2 Walkthrough - Remove Nth Node From End of List - Medium #19
Lecture 116 Code - Remove Nth Node From End of List
Section 25: Linked list question: Odd Even linked list (Medium)
Lecture 117 Explanation - Odd Even Linked List
Lecture 118 Intuition - Odd Even Linked List
Lecture 119 Pseudocode Implementation - Odd Even Linked List
Lecture 120 Walkthrough - Odd Even Linked List
Lecture 121 Code - Odd Even Linked List
Section 26: Google Linked list question: Merge K sorted lists (Hard)
Lecture 122 Explanation - Merge K Sorted Lists - Hard #23
Section 27: Facebook Backtracking question: Subsets (Medium)
Lecture 123 Explanation - Subsets
Lecture 124 Cascading solution explanation - Subsets
Lecture 125 Cascading solution walkthrough - Subsets
Lecture 126 Backtracking Approach 2 explanation - Subsets
Lecture 127 Implementing the code
Section 28: Amazon Backtracking question: Letter Combination of a Phone Number (Medium)
Lecture 128 Explanation - Letter Combinations of a Phone Number
Lecture 129 Intuition - Letter Combinations of a Phone Number
Lecture 130 Walkthrough - Letter Combinations of a Phone Number
Lecture 131 Code - Letter Combinations of a Phone Number
Section 29: Uber Backtracking question: Combination Sum (Medium)
Lecture 132 Explanation the problem
Lecture 133 Intuition behind the problem
Lecture 134 Walkthrough over the pseudocode
Lecture 135 Implementing the code
Section 30: Bloomberg Backtracking question: Palindrome Partitioning (Medium)
Lecture 136 Explaining the problem
Lecture 137 Pseudocode implementation
Lecture 138 Walkthrough over pseudocode
Lecture 139 Implementing the code
Section 31: Microsoft Trees question: Symmetric Trees (Easy)
Lecture 140 Explaining the problem
Lecture 141 Intuition behind the problem
Lecture 142 Walkthrough over pseudocode
Lecture 143 Implementing the code
Section 32: Google Trees question: Maximum Depth of a Binary Tree (Easy)
Lecture 144 Explaining the problem
Lecture 145 Intuition and pseudocode implementation
Lecture 146 Walkthrough over pseudocode
Lecture 147 Implementing the code
Section 33: Amazon Trees question: Path Sum (Easy)
Lecture 148 Explaining the problem
Lecture 149 Intuition behind the problem
Lecture 150 Walkthrough over pseudocode
Lecture 151 Coding the solution
Section 34: Facebook Trees question: Lowest Common Ancestor of a Binary Tree (Medium)
Lecture 152 Explaining the problem
Lecture 153 Intuition behind the problem
Lecture 154 Pseudocode implementation
Lecture 155 Walkthrough over pseudocode
Section 35: Google Trees question: Kth Smallest Element In a BST (Medium)
Lecture 156 Explaining the problem
Lecture 157 Optimized Solution Explanation- Kth Smallest Element in a BST - Medium #230
Lecture 158 Code - Kth Smallest Element in a BST - Medium #230
Section 36: Microsoft Trees question: Serialise And Deserialise Binary Tree (Hard)
Lecture 159 Explaining the "Serialisation"
Lecture 160 Walkthrough over pseudocode (Serialisation)
Lecture 161 Explaining the "Deserialisation"
Lecture 162 Walkthrough over pseudocode (Deserialisation)
Section 37: Microsoft Trees question: Binary Tree Maximum Path Sum (Hard)
Lecture 163 Explaining the problem
Lecture 164 Intuition behind the problem
Lecture 165 Walkthrough over pseudocode
Lecture 166 Coding the solution
Section 38: Google Stack Question: Min Stack (Easy)
Lecture 167 Brute force explanation - Min Stack - Easy #155
Lecture 168 Walkthrough over pseudocode
Lecture 169 Optimal solution explanation
Section 39: Amazon Stack Question: Valid Parenthesis (Easy)
Lecture 170 Explaining the problem
Lecture 171 Intuition behind this problem
Lecture 172 Pseudocode Implementation
Lecture 173 Walkthrough over the pseudocode
Lecture 174 Code - Valid Parenthesis - Easy #20
Section 40: Apple Stack Question: Binary Tree Level Order Traversal (Medium)
Lecture 175 Explaining the problem
Lecture 176 Walkthrough over pseudocode
Lecture 177 Implementing the code
Section 41: Microsoft Queue Question: Binary Tree Zigzag Level Order Traversal (Medium)
Lecture 178 Explaining the problem
Lecture 179 Intuition behind the problem
Lecture 180 Walkthrough over pseudocode
Lecture 181 Optimal solution explanation
Lecture 182 Optimal solution pseudocode walkthrough
Lecture 183 Implementing the code
Section 42: Stack Question: Binary Tree Postorder Traversal (Medium)
Lecture 184 Explanation of the problem
Lecture 185 Implementing the code
Section 43: Google Dynamic Programming Question: House Robber (Easy)
Lecture 186 Explanation behind the problem
Lecture 187 Intuition behind the problem
Lecture 188 2nd Approach: Bottom Up dynamic programming
Lecture 189 Walkthrough behind pseudocode
Lecture 190 Implementing the code
Section 44: Facebook Dynamic Programming Question: Best Time To Buy And Sell Stocks (Easy)
Lecture 191 Explanation behind the problem
Lecture 192 Intuition behind the problem
Lecture 193 Walkthrough over pseudocode
Lecture 194 Optimal solution explanation
Lecture 195 Coding the solution
Section 45: Amazon Dynamic Programming Question: Climbing Stairs (Easy)
Lecture 196 Explaining the problem
Lecture 197 Intuition behind the problem
Lecture 198 Implementation the pseudocode
Lecture 199 Bottom up approach explanation
Lecture 200 Bottom up approach walkthrough - Climbing Stairs - Easy #70
Lecture 201 Bottom up optimization - Climbing Stairs - Easy #70
Lecture 202 Code - Climbing Stairs - Easy #70
Section 46: Google Dynamic Programming Question: Coin Change (Medium)
Lecture 203 Explaining the problem
Lecture 204 Intuition behind the problem
Lecture 205 Pseudocode Implementation and optimisation
Lecture 206 Bottom up approach explanation
Lecture 207 Implementing the code
Section 47: Bloomberg Dynamic Programming Question: Unique Paths (Medium)
Lecture 208 Explaining the problem
Lecture 209 Pseudocode Implementation and walkthrough
Lecture 210 Implementing the code
Section 48: Microsoft Dynamic Programming Question: Longest Palindromic Substring (Medium)
Lecture 211 Explanation of the problem
Lecture 212 Initial Intuition behind the problem
Lecture 213 Optimising the previous solution
Lecture 214 Pseudocode Implementation
Lecture 215 Walkthrough over pseudocode
Lecture 216 Implementing the code
Section 49: Amazon Dynamic Programming Question: Trapping Rain Water (Hard)
Lecture 217 Explaining the problem
Lecture 218 Implementing the solution
Developers eager to pass the coding interview at huge companies like Google, Facebook, Microsoft, Amazon, etc,People who want to develop their problem solving skills,Developers getting ready for their interviews,Students getting ready for their internship interviews
TO MAC USERS: If RAR password doesn't work, use this archive program:
RAR Expander 0.8.5 Beta 4 and extract password protected files without error.
TO WIN USERS: If RAR password doesn't work, use this archive program:
Latest Winrar and extract password protected files without error.
